I started coding when I was still in grade school (primary) using the Atari 800XL that our father brought from overseas in the 80s. It had a BASIC language interpreter which would greet you by default after booting up the system. The 800XL also had a game cartridge slot where you could insert your Atari game cartridges to play games. While playing games like Missile Command, Defender, and Pacman was extremely fun, I found coding with it to be even more exciting. Being able to type instructions to the computer and get it to execute whatever you want, of course making sure that the syntax is correct, opened up a whole new world for me and was the start of my love for coding.
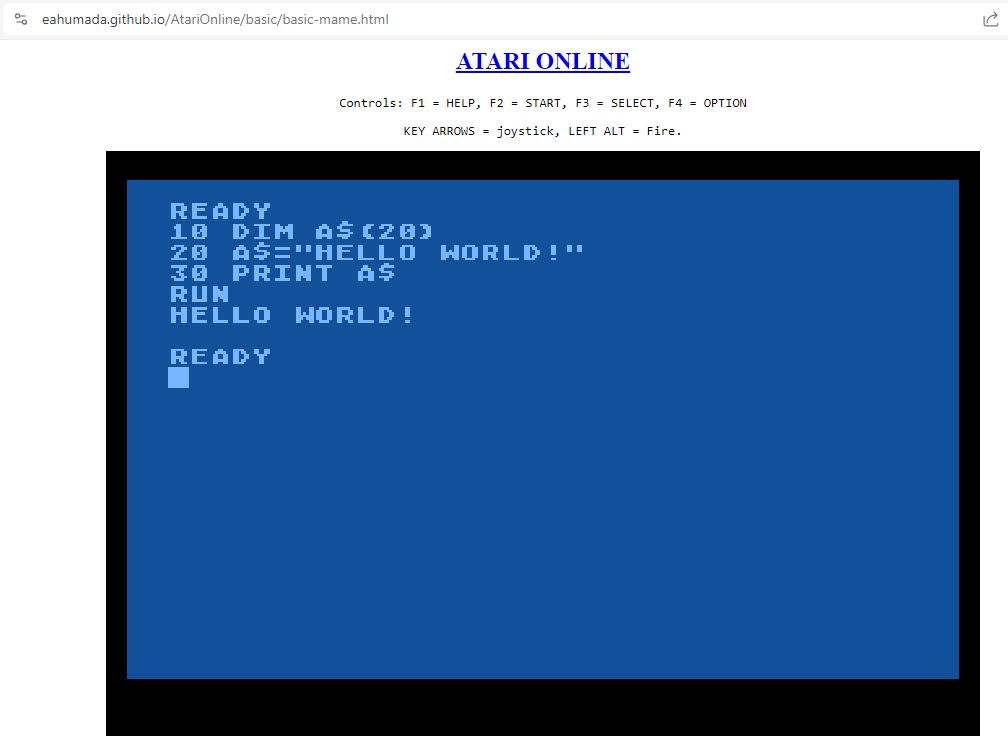
Recently the tech world, and coders especially, went abuzz when the CEO of Nvidia Jensen Huang remarked that kids of this generation need not learn to code. He argues that as AI systems become ever more advanced, coding tasks performed by humans will no longer be necessary. I am witnessing this myself and agree with Jensen that future AIs will be able to handle these tasks with ease, and it may not even matter what coding language the AI uses, or if coding languages are even still necessary as AI can write directly in machine code or to memory. However, I still teach my kids to code because I truly believe that the process of coding is not just about writing programs following the syntax of a given language, but allows you to be creative and think algorithmically—something that I believe will not change even with the advent of Generative AI. The future of coding may instead be replaced by writing LLM prompts, whose responses will depend on how well you wrote them, just like how you need to properly structure your code in order for the computer to give you the results that you want.
To give a simple coding example using the BASIC language that I played with as a kid, getting the computer to display the message “Hello World!” on the screen requires this simple line of code:
PRINT “Hello World!”
While this code is rather straightforward, it requires you to know the correct command and proper syntax for it to execute. So what if you do not know the commands and syntax for a programming language that you want to use? You can Google it and read through tons of advice from various coding forums on the web, or you can just ask ChatGPT “Write a BASIC code to print ‘Hello World!’” and you will get:
Here’s the BASIC code to print “Hello World!”: PRINT “Hello World!”
You can then copy the code to your code editor and interpreter and execute it. You can basically do the same for most programming languages as easily as you can type in English.
For a fun project, I asked the AI assistant Claude this: “Write a python code to emulate a BASIC programming language that is capable of interpreting the following commands: DIM, PRINT, CLS. It should also be able to accept multi-line code using line numbers and store values into string variables when issuing the DIM command. It should handle syntax errors for the above commands as well.”
Claude generated an impressive Python program that does exactly what I asked. I encourage you to try it out for yourself and have fun coding with AI language models! The future of programming in the age of AI is certainly exciting.
# -*- coding: utf-8 -*-
"""
Created on Sun Apr 14 21:11:25 2024
@author: makoantz.xyz
Python BASIC Interpreter V1.0
"""
import os
class BasicInterpreter:
def __init__(self):
self.variables = {}
def run(self):
while True:
self.lines.clear()
print("\nPython BASIC Interpreter")
print("(c)2024 https://makoantz.xyz")
print("Type 'RUN' to execute the code")
print("Type 'EXIT' to quit\n")
print("READY")
while True:
line = input('> ').strip()
if line.upper() == 'RUN':
self.execute_code()
break
elif line.upper() == 'EXIT':
return
elif line:
try:
line_number, command = line.split(' ', 1)
self.lines[int(line_number)] = command
except ValueError:
print(
"Syntax error: Line number missing or invalid"
)
def execute_code(self):
line_numbers = sorted(self.lines.keys())
for line_number in line_numbers:
command = self.lines[line_number]
try:
self.execute_command(command)
except SyntaxError as e:
print(f"Syntax error: {str(e)}")
def execute_command(self, command):
tokens = command.split()
keyword = tokens[0].upper()
try:
if keyword == 'DIM':
self.execute_dim(tokens[1:])
elif keyword == 'PRINT':
self.execute_print(tokens[1:])
elif keyword == 'CLS':
self.execute_cls()
elif '=' in command:
self.execute_assignment(command)
else:
raise SyntaxError(f"Invalid command: {keyword}")
except (IndexError, ValueError, KeyError):
raise SyntaxError(
f"Syntax error in command: {command}"
)
def execute_dim(self, tokens):
for token in tokens:
variable = token.split('$')[0]
self.variables[variable] = ''
def execute_print(self, tokens):
output = []
for token in tokens:
if token.startswith('"') and token.endswith('"'):
output.append(token[1:-1])
elif token in self.variables:
output.append(self.variables[token])
else:
raise SyntaxError(
f"Invalid argument in PRINT command: {token}"
)
print(' '.join(output))
def execute_cls(self):
os.system('cls' if os.name == 'nt' else 'clear')
def execute_assignment(self, command):
variable, value = command.split('=', 1)
variable = variable.strip()
value = value.strip()
if value.startswith('"') and value.endswith('"'):
self.variables[variable] = value[1:-1]
else:
raise SyntaxError(f"Invalid assignment: {command}")
def main():
interpreter = BasicInterpreter()
interpreter.run()
if __name__ == '__main__':
main()
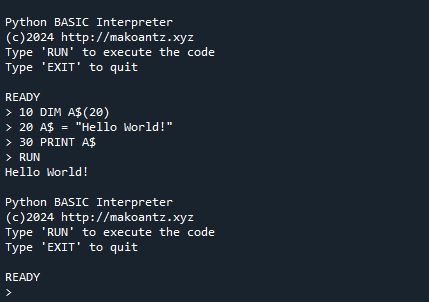
Leave a Reply